1. How does Java support the concept of encapsulation?
——————————————————————–
Save your time - order a paper!
Get your paper written from scratch within the tight deadline. Our service is a reliable solution to all your troubles. Place an order on any task and we will take care of it. You won’t have to worry about the quality and deadlines
Order Paper Now2. Describe the difference between an object and a class.
——————————————————————–
3. What is the difference between the contents of a Java variable
of a primitive type and a Java variable of a class type?
——————————————————————–
4. (a) How is a ‘static’ class method different from a regular
(non-static) class method?
(b) How is a ‘static’ variable in a class different from a
regular (instance) variable in a class?
——————————————————————–
5. What does the Java keyword ‘this’ refer to?
——————————————————————–
6. What does the Java ‘new’ operator do?
——————————————————————–
7. When does a class require an ‘equals’ method to be written?
——————————————————————–
8. What is method “overriding”? Why would you override a method?
——————————————————————–
9. What advantages does inheritance provide in Java?
——————————————————————–
10. What is dynamic binding? What advantage does it provide?
——————————————————————–
11. How is it possible for two String objects with identical values
not to be equal when compared with the == operator?
——————————————————————–
12. Why can a class variable of a base type be set to reference an
object of a derived type, eg:
Shape s = new Circle(“Blue”, 1.0);
——————————————————————–
13. How can the methods of a derived class access private instance
variables of its base class?
——————————————————————–
14. The following try/catch has code to explicitly handle any
IllegalArgumentException thrown. Show the modified code that
will also catch any other type of exception that could be thrown
by code called within the try block.
try
{
… methods called here …
…
}
catch (IllegalArgumentException e)
{
… code to handle IllegalArgumentException …
}
——————————————————————–
15. How is the caller’s flow of control (sequence of statement
execution) different when an exception is thrown from a method
that it calls, vs. when the method returns normally?
——————————————————————-
The following questions are 20 points each. Paste your source code
below each question, and example input and output. Do not include
the code required to test the methods, etc.
——————————————————————–
P1. Write a static method that checks if a String represents a valid
integer value and returns true if valid, or false if not. For
example, “123”, “-45”, and “0” are valid integers, but “x7” and
“3.14” are not. Use java.lang.Integer.parseInt() to perform the
actual validity checking inside your method. Your method should
also return false if the parameter String is null or empty.
It should NOT throw any exceptions back to the caller (but may
use exceptions internally).
Use the following prototype for your method:
public static boolean isValidInt(String testIntval)
Show example output for the following values entered:
“42”
“-123”
“12-34”
“10.5”
“x7”
“” (length 0 string)
null (pass a Java null reference in a hardcoded call to isValidInt)
——————————————————————–
P2. Write a Java method that returns the largest value passed to it.
Use the following prototype:
public static int max(int val1, int val2, int val3) {
// return the largest of the three values…
}
For example, the following call of your method sets ‘n’ to 30:
int n = max(20, 30, 10);
Note that any three values can be passed to this method.
The following would also set ‘n’ to 30:
int n = max(30, 30, 10);
Write a main() method to test your max method. It should input
three values, call your max method, and then print the three
values and returned max value as output, eg:
Input values: 30 20 10 – max value is 30
The max() method cannot read any input values, nor print any
output values. All input and printing is done by the main()
method that calls max().
Paste in both your source code for your max() method, and
the runtime output showing the parameter values and returned
maximum value for the following sample input values:
a) 30, 30, 30
b) 10, -10, 20
c) 40, 60, 50
d) 90, 70, 80
e) 50, 50, 25
f) 50, 50, 75
g) 200, 100, 200
h) 200, 400, 200
i) 300, 500, 500
j) 700, 500, 500
"If this is not the paper you were searching for, you can order your 100% plagiarism free, professional written paper now!"
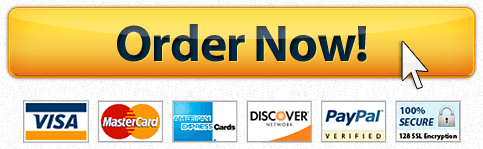
