Process and threads | Computer Science homework help
For this first assignment, you won’t be writing code; instead, you will be reading and executing the given below codes. Read the README instruction below for directions on how to compile and link the programs. Submit your answer in writing onto Blackboard.
- Experiment 1. Thread and Process Creation
Study the programs thr_create.c and fork.c. These programs measure the average time to create a thread using thr_create() and to create a process using fork(). Compile and execute the programs. What do you conclude from this experiment? Describe the reasons for the difference in timings. - Experiment 2. Processes vs Threads
Study the two programs thr_shared.c and proc_shared.c. These programs are identical except that one uses threads and the other uses processes. Compile and execute the programs. What do you conclude from this second experiment? Explain the reason for the difference in behavior. - Experiment 3: The Update Problem
Study the programs shared_data.c and SharedData.java. In these examples, multiple threads are both updating (i.e., modifying) a shared variable. Compile and execute both. Are the programs behaving “correctly”? What do you conclude from this experiment?
README Instructions - Run the program on Linux platform.
- To compile and run a C program (e.g., thr_create.c):
gcc -o thr_create thr_create.c -lpthread thr_create - To build and run a classfile from the java code: javac SharedData.java
java SharedData
Submission Guidelines and Requirements
Save your time - order a paper!
Get your paper written from scratch within the tight deadline. Our service is a reliable solution to all your troubles. Place an order on any task and we will take care of it. You won’t have to worry about the quality and deadlines
Order Paper Now- Submit your answers for all the experiments as a PDF file onto Blackboard
- Include your name, UCM ID and Certification statement in your solution:
//Your name
// Your UCM ID
//Certificate of Authenticity: “I certify that the codes/answers of this assignment are entirely my own work.”
thr_create.c
#include <sys/time.h> #include <stdio.h> #include <pthread.h> #include <errno.h> main()
{
struct timeval start,end; long forktime;
double avgtime; pthread_t last_thread; int i;
int iters = 250;
void *null_proc();
gettimeofday(&start,NULL);
for (i = 0; i < iters – 1; i++) /* create (iters-1) threads */ pthread_create(&last_thread,NULL,null_proc,NULL);
pthread_create(&last_thread,NULL,null_proc,NULL); /* create last thread */ gettimeofday(&end,NULL);
pthread_join(last_thread, NULL); /* wait for last thread */ forktime = (end.tv_sec – start.tv_sec)*1000000 +
(end.tv_usec – start.tv_usec); avgtime = (double)forktime/(double)iters;
printf(“Avg thr_create time = %f microsec\n”, avgtime); }
void *null_proc() {}
for (i = 0; i < iters; i++) /* create iters processes */ {
if ((pid = fork()) == 0) /* child process */ {
null_proc();
exit(1); }
else if (pid == -1) {
/* error */
printf(“error %d\n”,errno);
exit(-1); }
else /* parent process */ continue;
}
/* only parent process reaches this point */ gettimeofday(&end,NULL);
for ( i = 0; i < iters; i++) /* have to do a wait for each child */ wait(&status);
forktime = (end.tv_sec – start.tv_sec)*1000000 +
(end.tv_usec – start.tv_usec); avgtime = (double)forktime/(double)iters;
printf(“Avg fork time =%f microsec\n”, avgtime);
} null_proc() {
}
fork.c
#include <sys/time.h> #include <stdio.h> #include <errno.h>
main() {
int pid;
struct timeval start,end;
int i;
long forktime;
double avgtime;
int iters = 250;
int status; gettimeofday(&start,NULL);
#include <sys/time.h> #include <stdio.h> #include <pthread.h> #include <unistd.h> #include <stdlib.h>
void *proc();
int shared_number;
main() {
int i;
pthread_t new_thread; int sleep_time;
int seed;
shared_number = 1;
printf(“Enter a positive integer for seed: “);
scanf(“%d”,&seed);
srand48(seed); /* initialize seed of random number stream */
/* thr_create(NULL,0,proc,NULL,0,&new_thread);*/ pthread_create(&new_thread,NULL,proc,NULL);
/* create new thread */
for (i = 0; i < 50; i++) {
printf(“MAIN THREAD: i = %d, shared_number = %d\n”,i,shared_number); sleep_time = 100000.0*drand48(); /* generate random sleep time */ printf(“sleep time = %d microseconds\n”,sleep_time);
usleep(sleep_time);
shared_number = shared_number + 2; }
pthread_join(new_thread,NULL);
printf(“MAIN THREAD: DONE\n”); }
thr_shared.c
void *proc() {
int i = 0; int DONE;
DONE = 0; while (!DONE)
{ i++;
if (i%10000 == 0)
printf(“CHILD THREAD: i = %d,shared_number = %d\n”,i,shared_number);
if (i > 5000000) DONE = 1;
}
printf(“CHILD THREAD: DONE\n”);
}
#include <sys/time.h> #include <stdio.h> #include <pthread.h> #include <unistd.h> #include <stdlib.h> #include <errno.h>
void *proc();
int shared_number;
main() {
int i;
pthread_t new_thread; int sleep_time;
int pid;
int status;
int seed;
shared_number = 1;
proc_shared.c
printf(“Enter a positive integer for seed: “); scanf(“%d”,&seed);
srand48(seed);
if ((pid = fork()) == 0) {
proc();
exit(0); }
else if (pid == -1) {
printf(“error %d\n”,errno);
exit(-1); }
else
{ /* parent process */
for (i = 0; i < 50; i++) {
/* initialize random number stream */ /* child process */
/* error */
printf(“MAIN PROCESS: i = %d, shared_number = %d\n”,i,shared_number); sleep_time = 100000.0*drand48(); /* generate random sleep time */ printf(“sleep time = %d microseconds\n”,sleep_time);
usleep(sleep_time);
shared_number = shared_number + 2; }
wait(&status); /* wait for child process */
printf(“MAIN PROCESS: DONE\n”); }
}
void *proc() {
int i;
int DONE;
DONE = 0;
i = 0;
while (!DONE)
{ i++;
if (i%10000 == 0)
printf(“CHILD PROCESS: i = %d,shared_number = %d\n”,i,shared_number); if (i > 5000000)
DONE = 1; }
printf(“CHILD PROCESS: DONE\n”); }
shared_data.c
#include <sys/time.h> #include <stdio.h> #include <pthread.h> #include <unistd.h> #include <stdlib.h>
void *proc();
int shared_number;
main() {
int i;
pthread_t new_thread; int sleep_time;
int number;
int seed;
shared_number = 1;
printf(“Enter a positive integer for seed: “); scanf(“%d”,&seed);
srand48(seed);
pthread_create(&new_thread,NULL,proc,NULL);
for (i = 0; i < 20; i++) {
number = shared_number;
printf(“MAIN THREAD: i = %d, shared_number = %d\n”,i,shared_number); sleep_time = 100000.0*drand48(); /* generate random sleep time */ printf(“sleep time = %d microseconds\n”,sleep_time);
usleep(sleep_time);
shared_number = number + 2; }
pthread_join(new_thread,NULL);
printf(“MAIN THREAD: DONE\n”); }
void *proc() {
int number,i; int sleep_time;
printf(“CHILD\n”); for (i = 0; i < 10; i++)
{
number = shared_number;
printf(“CHILD THREAD: i = %d, shared_number = %d\n”,i,shared_number); sleep_time = 100000.0*drand48(); /* generate random sleep time */ printf(“sleep time = %d microseconds\n”,sleep_time);
usleep(sleep_time);
shared_number = number + 200000;
}
printf(“CHILD THREAD: DONE\n”);
}
SharedData.java
import java.util.Random;
public class SharedData extends Thread {
static Random gRandom = new Random(); static int gSharedNumber = 0;
private int gID;
public SharedData(int id) {
this.gID = id; }
public void run() { for(int i=0;i<20;i++) {
int number = gSharedNumber; System.out.println(“SharedData[“+gID+”](i=”+i+”) = “+gSharedNumber); try { Thread.sleep(gRandom.nextInt(1000));
} catch (InterruptedException e) { /* ignore */ }
if(gID == 0) gSharedNumber = number + 1;
else gSharedNumber = number + 1000;
} }
public static void main(String args[]) throws Exception { SharedData sd1 = new SharedData(0);
SharedData sd2 = new SharedData(1);
sd1.start(); sd2.start();
sd1.join(); sd2.join(); }
}
"If this is not the paper you were searching for, you can order your 100% plagiarism free, professional written paper now!"
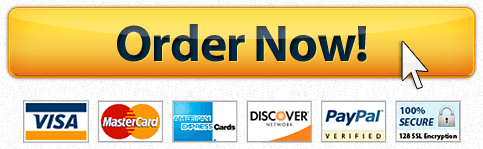
